To allocate more memory to PyTorch, you can increase the batch size of your data when training your neural network models. This allows PyTorch to utilize more memory during the training process. Additionally, you can try running your code on a machine with more RAM or GPU memory to provide PyTorch with more resources to work with. Another option is to optimize your code for memory efficiency by using techniques such as reducing unnecessary variable storage or using data loaders to load data in batches. Finally, you can adjust the memory limit configuration in PyTorch to allow it to use more memory when running your code.
Best Python Books to Read In November 2024
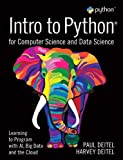
Rating is 4.9 out of 5
Intro to Python for Computer Science and Data Science: Learning to Program with AI, Big Data and The Cloud
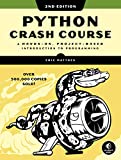
Rating is 4.8 out of 5
Python Crash Course, 2nd Edition: A Hands-On, Project-Based Introduction to Programming
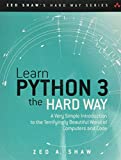
Rating is 4.7 out of 5
Learn Python 3 the Hard Way: A Very Simple Introduction to the Terrifyingly Beautiful World of Computers and Code (Zed Shaw's Hard Way Series)
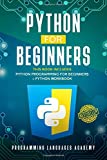
Rating is 4.6 out of 5
Python for Beginners: 2 Books in 1: Python Programming for Beginners, Python Workbook
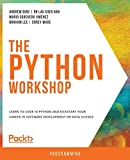
Rating is 4.5 out of 5
The Python Workshop: Learn to code in Python and kickstart your career in software development or data science
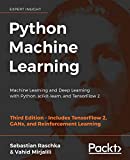
Rating is 3.9 out of 5
Python Machine Learning: Machine Learning and Deep Learning with Python, scikit-learn, and TensorFlow 2, 3rd Edition
What is the trade-off between memory allocation and computational efficiency in PyTorch?
The trade-off between memory allocation and computational efficiency in PyTorch is that optimizing for one can often lead to a decrease in the other.
When PyTorch allocates memory for storing tensors, it needs to strike a balance between conserving memory and ensuring that calculations are performed efficiently. This means that if PyTorch prioritizes minimizing memory usage, it may need to use more computational resources to manage the memory allocation and deallocation processes. On the other hand, if PyTorch prioritizes computational efficiency, it may end up using more memory than necessary, potentially leading to wastage.
In general, optimizing for memory allocation can help prevent out-of-memory errors and improve the overall performance of the PyTorch application. However, it is essential to be mindful of the potential impact on computational efficiency and adjust accordingly based on the specific requirements of the application. Ultimately, the trade-off between memory allocation and computational efficiency in PyTorch will depend on the specific use case and the balance that needs to be struck between these two factors.
How to allocate more GPU memory to PyTorch?
To allocate more GPU memory to PyTorch, you can use the following code snippet before creating your model or tensor:
1 2 3 4 5 6 7 |
import torch # set the desired GPU memory limit torch.backends.cudnn.benchmark = True torch.backends.cudnn.enabled = True torch.cuda.set_device(0) # specify GPU device if you have more than one GPU torch.cuda.set_per_process_memory_fraction(0.9) # allocate 90% of GPU memory |
Make sure to adjust the memory fraction value (0.9 in the above example) based on your needs and the available GPU memory on your system. This code will ensure that PyTorch allocates the specified percentage of GPU memory for your model or tensor.
What is the maximum memory allocation for PyTorch?
The maximum memory allocation for PyTorch depends on the specific hardware used. Generally, PyTorch can utilize all available memory on the GPU or CPU that it is running on. However, it is always recommended to allocate memory judiciously and avoid exceeding the available memory in order to prevent out-of-memory errors and crashes.