To calculate unique rows with values in Pandas, you can use the drop_duplicates() method. This method will return a new DataFrame with only the unique rows based on specified columns. You can also use the nunique() method to count the number of unique values in each column. Additionally, you can use the unique() method to return an array of unique values in a specified column. These methods can help you efficiently calculate unique rows with values in your Pandas DataFrame.
Best Python Books to Read In November 2024
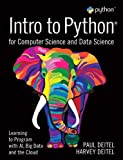
Rating is 4.9 out of 5
Intro to Python for Computer Science and Data Science: Learning to Program with AI, Big Data and The Cloud
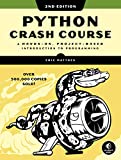
Rating is 4.8 out of 5
Python Crash Course, 2nd Edition: A Hands-On, Project-Based Introduction to Programming
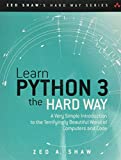
Rating is 4.7 out of 5
Learn Python 3 the Hard Way: A Very Simple Introduction to the Terrifyingly Beautiful World of Computers and Code (Zed Shaw's Hard Way Series)
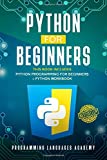
Rating is 4.6 out of 5
Python for Beginners: 2 Books in 1: Python Programming for Beginners, Python Workbook
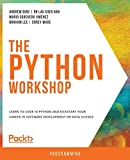
Rating is 4.5 out of 5
The Python Workshop: Learn to code in Python and kickstart your career in software development or data science
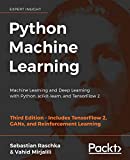
Rating is 3.9 out of 5
Python Machine Learning: Machine Learning and Deep Learning with Python, scikit-learn, and TensorFlow 2, 3rd Edition
How to filter out non-unique rows in pandas?
To filter out non-unique rows in a pandas DataFrame, you can use the duplicated()
function along with boolean indexing. Here's an example:
1 2 3 4 5 6 7 8 9 10 11 |
import pandas as pd # Create a sample DataFrame data = {'A': [1, 2, 3, 1, 2], 'B': ['foo', 'bar', 'foo', 'bar', 'baz']} df = pd.DataFrame(data) # Filter out non-unique rows unique_rows = df[~df.duplicated()] print(unique_rows) |
In this example, the duplicated()
function is used to identify duplicated rows in the DataFrame. By using the ~
operator along with boolean indexing, we can filter out the non-unique rows and store the unique rows in the unique_rows
variable.
What is the role of checking for duplicates within a specific column in pandas?
The role of checking for duplicates within a specific column in pandas is to identify and remove any redundant or repetitive data entries. This is important because duplicates can skew analyses and lead to inaccurate results. By checking for duplicates within a specific column, data cleanliness and accuracy can be ensured, thus improving the quality of the analysis and resulting insights derived from the data.
What is the effect of NaN values on counting unique rows in pandas?
Counting unique rows in pandas ignores NaN values. This means that if a row contains a NaN value in any column, it will still be considered unique when counting unique rows in pandas.
What is the use of generating a list of unique values from a dataframe in pandas?
Generating a list of unique values from a dataframe in pandas allows us to quickly identify and analyze the distinct values present in a particular column or series. This can be useful for data cleaning and preparation, as well as for gaining insights into the underlying data distribution and patterns. Unique value lists can also be used for further data manipulation tasks, such as grouping, filtering, or transforming the data.
How to calculate the number of unique values in each column in pandas?
You can calculate the number of unique values in each column of a pandas DataFrame by using the nunique() function. Here is an example:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
import pandas as pd # Creating a sample DataFrame data = {'A': [1, 2, 3, 2, 1], 'B': ['foo', 'bar', 'foo', 'bar', 'baz'], 'C': ['apple', 'orange', 'apple', 'banana', 'apple']} df = pd.DataFrame(data) # Calculating the number of unique values in each column unique_counts = df.nunique() print(unique_counts) |
Output:
1 2 3 4 |
A 3 B 3 C 3 dtype: int64 |
This will return a Series where the index represents the column names and the values represent the number of unique values in each column.