To get a value to a variable using wxPython, you can use the GetValue method on the specific wxPython control that you are working with. For example, if you are using a wx.TextCtrl, you can call the GetValue method on it to retrieve the text entered by the user and assign it to a variable. Similarly, for other controls like wx.Choice or wx.SpinCtrl, you can use their specific methods to get the selected value and assign it to a variable. This way, you can store the user input or selected value in a variable for further processing or use in your wxPython application.
Best Python Books to Read In May 2025
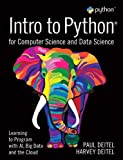
Rating is 4.9 out of 5
Intro to Python for Computer Science and Data Science: Learning to Program with AI, Big Data and The Cloud
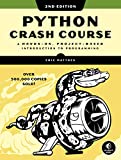
Rating is 4.8 out of 5
Python Crash Course, 2nd Edition: A Hands-On, Project-Based Introduction to Programming
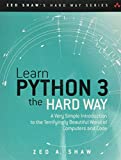
Rating is 4.7 out of 5
Learn Python 3 the Hard Way: A Very Simple Introduction to the Terrifyingly Beautiful World of Computers and Code (Zed Shaw's Hard Way Series)
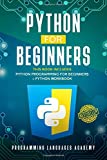
Rating is 4.6 out of 5
Python for Beginners: 2 Books in 1: Python Programming for Beginners, Python Workbook
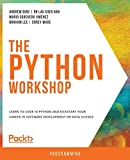
Rating is 4.5 out of 5
The Python Workshop: Learn to code in Python and kickstart your career in software development or data science
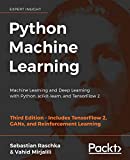
Rating is 3.9 out of 5
Python Machine Learning: Machine Learning and Deep Learning with Python, scikit-learn, and TensorFlow 2, 3rd Edition
What is a variable in wxPython?
In wxPython, a variable is a container used to store a value such as a number, string, or object. Variables are used to store and manipulate data in a program, making it easier to work with and reference values throughout the code. Variables in wxPython follow the same rules and conventions as variables in the Python programming language.
What is variable manipulation in wxPython?
Variable manipulation in wxPython refers to the process of changing the value of a variable within a wxPython application. This can involve tasks such as setting the value of a variable based on user input, modifying the value of a variable through calculations or operations, or updating the value of a variable in response to events or changes within the application. Variable manipulation is a common practice in wxPython programming to control the behavior and appearance of graphical user interfaces.
How to reset a variable in wxPython?
To reset a variable in wxPython, you can simply assign a new value to the variable. For example:
1 2 3 4 5 6 7 |
# Define a variable my_variable = 10 # Reset the variable by assigning a new value my_variable = 0 print(my_variable) # Output: 0 |
Alternatively, if you want to reset a variable to its initial value, you can save the initial value in a separate variable and assign it back to the original variable when needed. For example:
1 2 3 4 5 6 7 8 9 10 |
# Define the initial value of the variable initial_value = 10 # Define the variable my_variable = initial_value # Reset the variable to its initial value my_variable = initial_value print(my_variable) # Output: 10 |
How to assign a variable in wxPython?
In wxPython, you can assign a variable using the standard Python syntax. Simply use the variable name followed by the assignment operator "=", and then the value you want to assign to the variable. Here is an example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
import wx app = wx.App() frame = wx.Frame(None, title="Hello, wxPython") panel = wx.Panel(frame) # Assign a value to a variable my_variable = "Hello, World!" # Access and use the variable later in the code print(my_variable) frame.Show() app.MainLoop() |
In this example, the variable my_variable
is assigned the value "Hello, World!". Later in the code, we can access and use the variable as needed.