To pad a tensor with zeros in PyTorch, you can use the torch.nn.functional.pad
function. This function allows you to specify the padding size for each dimension of the tensor. You can pad the tensor with zeros before or after the data in each dimension. Padding a tensor with zeros can be useful when you want to ensure that the input tensor has a specific shape or size before passing it to a neural network.
Best Python Books to Read In October 2024
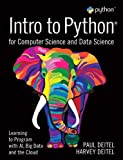
Rating is 4.9 out of 5
Intro to Python for Computer Science and Data Science: Learning to Program with AI, Big Data and The Cloud
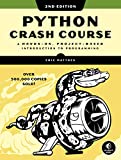
Rating is 4.8 out of 5
Python Crash Course, 2nd Edition: A Hands-On, Project-Based Introduction to Programming
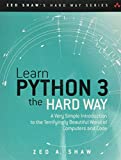
Rating is 4.7 out of 5
Learn Python 3 the Hard Way: A Very Simple Introduction to the Terrifyingly Beautiful World of Computers and Code (Zed Shaw's Hard Way Series)
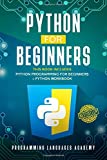
Rating is 4.6 out of 5
Python for Beginners: 2 Books in 1: Python Programming for Beginners, Python Workbook
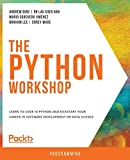
Rating is 4.5 out of 5
The Python Workshop: Learn to code in Python and kickstart your career in software development or data science
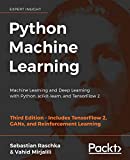
Rating is 3.9 out of 5
Python Machine Learning: Machine Learning and Deep Learning with Python, scikit-learn, and TensorFlow 2, 3rd Edition
What is the purpose of padding a tensor with zeros in PyTorch?
Padding a tensor with zeros in PyTorch is a common technique used in neural networks for image processing tasks such as convolutional operations. The purpose of padding is to add additional zeros around the edges of the input tensor, creating a border of zeros around the original data.
Padding with zeros helps maintain the spatial dimensions of the input tensor when applying convolutional operations, which can help prevent loss of information at the edges of the image. It also helps preserve the size of the output tensor after convolutional operations, ensuring that the output tensor has the same dimensions as the input tensor.
Overall, padding with zeros in PyTorch is used to ensure that the spatial information in the input data is preserved and to help make convolutional operations more effective.
How to pad a tensor with zeros in PyTorch using torch.nn.functional.pad?
You can pad a tensor with zeros using the torch.nn.functional.pad
function in PyTorch. Here is an example code snippet demonstrating how to pad a tensor with zeros using this function:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
import torch import torch.nn.functional as F # Create a tensor tensor = torch.tensor([[1, 2], [3, 4]]) # Specify the padding values for each dimension padding = (1, 2, 1, 2) # (left, right, top, bottom) # Pad the tensor with zeros padded_tensor = F.pad(tensor, padding, mode='constant', value=0) print("Original Tensor:") print(tensor) print("Padded Tensor:") print(padded_tensor) |
In this code snippet:
- We create a tensor tensor with values [1, 2] and [3, 4].
- We define the padding values as (1, 2, 1, 2), which means that we want to pad the tensor with 1 zero element on the left, 2 zero elements on the right, 1 zero element on the top, and 2 zero elements on the bottom.
- We use the F.pad function to pad the tensor with zeros, specifying the mode='constant' parameter to pad with a constant value (zero) and setting the value=0 parameter to specify that we want to pad with zeros.
- Finally, we print the original tensor and the padded tensor to see the results.
This code will output the following:
1 2 3 4 5 6 7 8 |
Original Tensor: tensor([[1, 2], [3, 4]]) Padded Tensor: tensor([[0, 0, 0, 0], [0, 1, 2, 0], [0, 3, 4, 0], [0, 0, 0, 0]]) |