To remove empty lists in pandas, you can use the dropna()
method along with the apply()
function.
First, identify the columns that contain lists as values using the applymap(type)
function.
Next, drop the rows with empty lists using applymap(len)
to get the length of each list and then using dropna()
to remove rows where the length is 0.
Finally, you can use df.reset_index(drop=True)
to reset the index after removing the empty lists.
Best Python Books to Read In November 2024
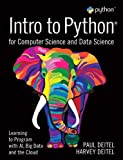
Rating is 4.9 out of 5
Intro to Python for Computer Science and Data Science: Learning to Program with AI, Big Data and The Cloud
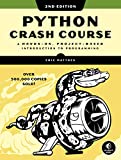
Rating is 4.8 out of 5
Python Crash Course, 2nd Edition: A Hands-On, Project-Based Introduction to Programming
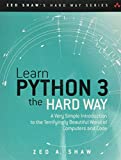
Rating is 4.7 out of 5
Learn Python 3 the Hard Way: A Very Simple Introduction to the Terrifyingly Beautiful World of Computers and Code (Zed Shaw's Hard Way Series)
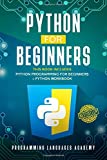
Rating is 4.6 out of 5
Python for Beginners: 2 Books in 1: Python Programming for Beginners, Python Workbook
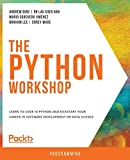
Rating is 4.5 out of 5
The Python Workshop: Learn to code in Python and kickstart your career in software development or data science
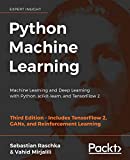
Rating is 3.9 out of 5
Python Machine Learning: Machine Learning and Deep Learning with Python, scikit-learn, and TensorFlow 2, 3rd Edition
What is the function to remove empty list in pandas?
You can use the dropna() function in pandas to remove empty lists or NaN values from a DataFrame. Here is an example of how you can use this function:
1 2 3 4 5 6 7 8 9 10 11 |
import pandas as pd # Create a DataFrame with some empty lists data = {'col1': [[], [1, 2, 3], [], [4, 5]], 'col2': [1, 2, 3, 4]} df = pd.DataFrame(data) # Remove empty lists from the 'col1' column df['col1'] = df['col1'].apply(lambda x: x if x != [] else None) df.dropna(inplace=True) print(df) |
This will remove the rows with empty lists in the 'col1' column from the DataFrame.
What is the syntax to drop rows with empty list in pandas?
To drop rows with empty lists in Pandas, you can use the following syntax:
1
|
df = df[df['column_name'].apply(lambda x: len(x) > 0)]
|
This code snippet will filter the DataFrame df
based on the length of the lists in the column named 'column_name'
. Rows with empty lists will be removed from the DataFrame.
How to drop empty list in pandas dataframe?
If you want to drop rows in a pandas dataframe where all the values are NaN or empty, you can use the following code:
1
|
df.dropna(how='all', inplace=True)
|
This will drop any rows where all the values are NaN or empty. The inplace=True
parameter will make the changes to the original dataframe.