In order to extract data from a dictionary within a pandas dataframe, you can access the dictionary values using the apply()
function along with a lambda function. First, you need to create a new column in the dataframe to store the dictionary values. Then, you can use the apply()
function to extract the values from the dictionary by providing the key as an argument to the lambda function. This will return the specific value associated with that key in the dictionary for each row in the dataframe.
Best Python Books to Read In November 2024
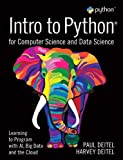
Rating is 4.9 out of 5
Intro to Python for Computer Science and Data Science: Learning to Program with AI, Big Data and The Cloud
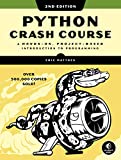
Rating is 4.8 out of 5
Python Crash Course, 2nd Edition: A Hands-On, Project-Based Introduction to Programming
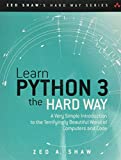
Rating is 4.7 out of 5
Learn Python 3 the Hard Way: A Very Simple Introduction to the Terrifyingly Beautiful World of Computers and Code (Zed Shaw's Hard Way Series)
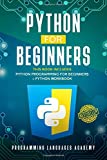
Rating is 4.6 out of 5
Python for Beginners: 2 Books in 1: Python Programming for Beginners, Python Workbook
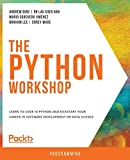
Rating is 4.5 out of 5
The Python Workshop: Learn to code in Python and kickstart your career in software development or data science
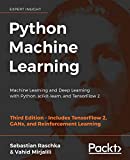
Rating is 3.9 out of 5
Python Machine Learning: Machine Learning and Deep Learning with Python, scikit-learn, and TensorFlow 2, 3rd Edition
Is it possible to extract nested dictionary data from a pandas dataframe?
Yes, it is possible to extract nested dictionary data from a pandas dataframe. One way to do this is by using the json_normalize
function from the pandas.io.json
module. This function can be used to flatten the nested dictionary data into a pandas dataframe.
How to extract specific dictionary keys from a pandas dataframe column?
You can extract specific dictionary keys from a pandas dataframe column by using the apply
function along with a lambda function to extract the specific keys. Here's an example:
1 2 3 4 5 6 7 8 9 10 |
import pandas as pd # Create a sample dataframe df = pd.DataFrame({'data': [{'name': 'Alice', 'age': 30}, {'name': 'Bob', 'age': 25}]}) # Extract specific keys from the dictionary in the 'data' column keys_to_extract = ['name'] df['extracted_keys'] = df['data'].apply(lambda x: {k: x.get(k) for k in keys_to_extract}) print(df) |
This code will create a new column called 'extracted_keys' in the dataframe df
that contains only the 'name' key from the dictionaries in the 'data' column. You can customize the keys_to_extract
list to extract other specific keys as needed.
What is the method to extract dictionary values from a pandas dataframe row?
You can extract dictionary values from a pandas dataframe row in Python by using the to_dict()
method. Here is an example:
1 2 3 4 5 6 7 8 9 |
import pandas as pd # Create a sample pandas dataframe data = {'A': [1, 2, 3], 'B': [{'key1': 'value1', 'key2': 'value2'}, {'key3': 'value3'}, {'key4': 'value4'}]} df = pd.DataFrame(data) # Extract dictionary values from a row row_values = df.iloc[1].to_dict() print(row_values) |
This code snippet creates a sample pandas dataframe with a column containing dictionaries as values. It then extracts the dictionary values from the second row and prints them.