To create a pandas dataframe from a list of dictionaries, you can simply use the pd.DataFrame() function and pass the list of dictionaries as an argument. Each dictionary in the list will become a row in the dataframe, with the keys of the dictionaries becoming the column names. This can be a quick and efficient way to convert structured data into a dataframe for further analysis and manipulation in Python using the pandas library.
Best Python Books to Read In November 2024
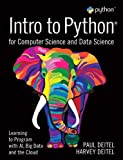
Rating is 4.9 out of 5
Intro to Python for Computer Science and Data Science: Learning to Program with AI, Big Data and The Cloud
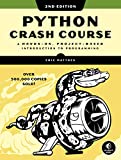
Rating is 4.8 out of 5
Python Crash Course, 2nd Edition: A Hands-On, Project-Based Introduction to Programming
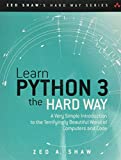
Rating is 4.7 out of 5
Learn Python 3 the Hard Way: A Very Simple Introduction to the Terrifyingly Beautiful World of Computers and Code (Zed Shaw's Hard Way Series)
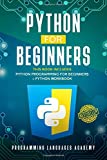
Rating is 4.6 out of 5
Python for Beginners: 2 Books in 1: Python Programming for Beginners, Python Workbook
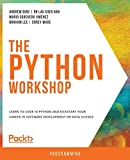
Rating is 4.5 out of 5
The Python Workshop: Learn to code in Python and kickstart your career in software development or data science
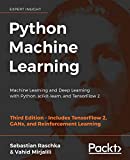
Rating is 3.9 out of 5
Python Machine Learning: Machine Learning and Deep Learning with Python, scikit-learn, and TensorFlow 2, 3rd Edition
What is the purpose of the reset_index method in pandas dataframes?
The purpose of the reset_index
method in pandas dataframes is to reset the index of the dataframe. This method can be useful when the original index needs to be reset or if the index needs to be converted to a column in the dataframe. By using this method, the current index of the dataframe is reset to the default integer index starting from 0, and a new default index column is added to the dataframe. This can be helpful for data manipulation and analysis, as it allows for easier slicing, merging, and reshaping of the dataframe.
How to perform arithmetic operations on columns in a pandas dataframe?
To perform arithmetic operations on columns in a pandas dataframe, you can use the following steps:
- Create a pandas dataframe:
1 2 3 4 5 6 |
import pandas as pd data = {'A': [1, 2, 3, 4], 'B': [5, 6, 7, 8]} df = pd.DataFrame(data) print(df) |
- Perform arithmetic operations on columns:
You can perform arithmetic operations such as addition, subtraction, multiplication, and division on columns using the following syntax:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
# Addition df['C'] = df['A'] + df['B'] # Subtraction df['D'] = df['A'] - df['B'] # Multiplication df['E'] = df['A'] * df['B'] # Division df['F'] = df['B'] / df['A'] print(df) |
This will add new columns 'C', 'D', 'E', and 'F' to the dataframe with the results of the arithmetic operations performed on columns 'A' and 'B'.
- Use the result for further analysis or visualization.
You can now use the result of the arithmetic operations for further analysis, visualization, or any other data processing tasks in your pandas dataframe.
How to rename columns in a pandas dataframe?
You can rename columns in a pandas dataframe using the rename
method. Here's an example:
1 2 3 4 5 6 7 8 9 10 |
import pandas as pd # Create a sample dataframe data = {'A': [1, 2, 3], 'B': [4, 5, 6]} df = pd.DataFrame(data) # Rename columns df = df.rename(columns={'A': 'X', 'B': 'Y'}) print(df) |
This will output:
1 2 3 4 |
X Y 0 1 4 1 2 5 2 3 6 |
In this example, we used the rename
method to rename columns 'A' to 'X' and 'B' to 'Y' in the dataframe. The columns
parameter is a dictionary where keys are the old column names and values are the new column names.