To use lambda with pandas correctly, you can pass a lambda function directly to one of the pandas methods that accept a function as an argument. This can be useful when you want to apply a custom operation to each element in a column or row of a DataFrame. For example, you can use the apply
method with a lambda function to transform the values in a column based on some logic. Additionally, you can use the map
method with a lambda function to apply a custom operation to each element in a Series. Just remember to keep your lambda functions simple and clear to ensure readability and maintainability.
Best Python Books to Read In November 2024
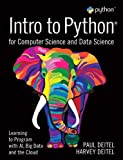
Rating is 4.9 out of 5
Intro to Python for Computer Science and Data Science: Learning to Program with AI, Big Data and The Cloud
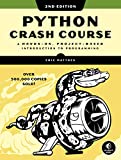
Rating is 4.8 out of 5
Python Crash Course, 2nd Edition: A Hands-On, Project-Based Introduction to Programming
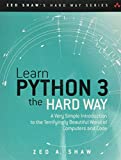
Rating is 4.7 out of 5
Learn Python 3 the Hard Way: A Very Simple Introduction to the Terrifyingly Beautiful World of Computers and Code (Zed Shaw's Hard Way Series)
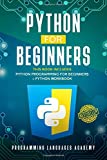
Rating is 4.6 out of 5
Python for Beginners: 2 Books in 1: Python Programming for Beginners, Python Workbook
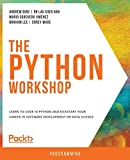
Rating is 4.5 out of 5
The Python Workshop: Learn to code in Python and kickstart your career in software development or data science
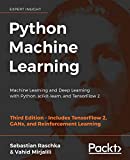
Rating is 3.9 out of 5
Python Machine Learning: Machine Learning and Deep Learning with Python, scikit-learn, and TensorFlow 2, 3rd Edition
What is the syntax for using lambda with pandas?
In pandas, you can use the apply()
function along with a lambda function to apply a custom function to each element of a DataFrame or Series.
The syntax for using lambda with pandas is as follows:
1 2 3 4 5 6 7 8 9 10 11 12 |
import pandas as pd # Create a DataFrame df = pd.DataFrame({ 'A': [1, 2, 3], 'B': [4, 5, 6] }) # Apply a lambda function to each element in the column 'A' df['A'] = df['A'].apply(lambda x: x*2) print(df) |
This will multiply each element in the 'A' column of the DataFrame by 2. You can replace the lambda function with any custom function that you want to apply to the DataFrame or Series.
What is the advantage of using lambda instead of regular functions in pandas?
Using lambda functions in pandas offers several advantages over regular functions:
- Conciseness: Lambda functions are typically shorter and more concise than regular functions, making them easier to write and understand.
- Readability: Lambda functions are often used in conjunction with functions like apply(), map(), filter() etc., making the code more readable and easier to interpret.
- Efficiency: Lambda functions are more lightweight and may offer better performance than regular functions, especially when working with large datasets in pandas.
- Avoiding unnecessary function creation: Lambda functions can be used inline without the need to define a separate function, making them convenient for quick and one-off operations.
- Flexibility: Lambda functions allow for quick customization and can be easily modified or adapted to suit specific requirements without the need to define a separate function.
How to use lambda function in pandas merge operation?
You can use lambda function in pandas merge operation by passing the lambda function as the on
argument in the merge
function. Here's an example:
1 2 3 4 5 6 7 8 9 10 11 12 |
import pandas as pd # create two dataframes df1 = pd.DataFrame({'key': ['A', 'B', 'C', 'D'], 'value1': [1, 2, 3, 4]}) df2 = pd.DataFrame({'key': ['A', 'B', 'E', 'F'], 'value2': [5, 6, 7, 8]}) # merge the two dataframes using a lambda function merged_df = pd.merge(df1, df2, on=lambda x: x['key'], how='inner') print(merged_df) |
In this example, the lambda function is used to specify the join key for the merge operation. The lambda function takes a dataframe as input and returns the column on which to join the dataframes. This allows you to perform more complex operations on the join key before merging the dataframes.